Introduction
In today’s fast-paced digital world, software-as-a-service (SaaS) applications are becoming increasingly popular. Building a SaaS dashboard from scratch can be a complex and time-consuming task. However, with the right tools and technologies, it is possible to streamline the development process and create a powerful and efficient dashboard. In this blog, we will explore building a SaaS dashboard with React and Firebase.
React is a JavaScript library developed by Facebook that allows for creating interactive user interfaces. On the other hand, Firebase is a serverless mobile and web application platform provided by Google. By combining these two powerful tools, we can create a dynamic and responsive SaaS dashboard that meets the needs of modern web applications. This tutorial will guide you through creating a SaaS dashboard by fetching data from Google Sheets using the Google Sheets API. We will also be using FusionCharts, a JavaScript-based charting library, and Bulma, a CSS framework based on Flexbox.
Setting the Stage for Your SaaS Dashboard
Before diving into the technical details, it’s important to understand the core objectives of your SaaS dashboard. A SaaS dashboard is a central hub where users can access and manage their account information, view analytics and reports, and perform various tasks related to the SaaS application. The dashboard should provide a seamless user experience and make it easy for users to find the information they need. Additionally, it should allow users to update their personal information, manage their subscriptions, and access any other features or functionality specific to the SaaS application.
Understanding the Core Objectives of Your SaaS Dashboard
One of the main objectives of a SaaS dashboard is to provide users with the necessary functionality to manage their accounts and perform various tasks. This includes features such as updating personal information, managing subscriptions, accessing support, and more. The dashboard should also provide users with analytics and reports to track their usage and monitor the performance of the SaaS application. This can include metrics such as user activity, revenue, and other key performance indicators. Finally, the dashboard should prioritize user experience by providing a clean and intuitive interface, easy navigation, and responsive design. It should be easy for users to find the information they need and perform tasks efficiently. By understanding these core objectives, you can plan and design your SaaS dashboard to meet the needs of your users.
Choosing the Right Tools: Why React and Firebase?
When building a SaaS dashboard, it’s essential to choose the right tools and technologies. React is a popular choice among developers for building user interfaces due to its component-based architecture, virtual DOM, and reusability. React allows for the creation of dynamic and responsive UI components, making it ideal for building complex dashboards.
Firebase, on the other hand, provides a powerful backend platform for SaaS applications. It offers a range of features such as authentication, real-time database, and hosting, making it a comprehensive solution for building scalable and feature-rich applications. Firebase’s real-time database allows for the synchronization of data across multiple clients in real time, making it ideal for collaborative applications. Additionally, Firebase’s authentication feature provides secure and easy-to-use user authentication, allowing users to sign in with various methods such as email, social media accounts, and more, ensuring the protection of user information.
By combining React and Firebase, developers can leverage the best of both worlds. React provides a robust framework for building user interfaces, while Firebase offers the necessary backend infrastructure and features to support a SaaS application’s core functionalities. Together, React and Firebase provide a scalable solution that can handle the demands of a growing user base, including the integration of Stripe for secure payment processing.
Check out an article on 11 SaaS Success Stories Worldwide with Real-World Examples.
Preparing Your Development Environment
Before we start building our SaaS dashboard, we need to set up our development environment. To do this, we will need to have Node.js installed on our machine. Node.js is a JavaScript runtime that allows us to run JavaScript code outside of a browser, making it ideal for building server-side applications. npm (Node Package Manager) is also bundled with Node.js and allows us to install and manage dependencies for our projects.
To set up your development environment, you will need to download and install Node.js from the official Node.js website. Once installed, you will have access to the npm command-line interface (CLI), which we will use to install the necessary dependencies for our SaaS dashboard project.
Installing Node.js and npm
To install Node.js, you will need to visit the official Node.js website and download the relevant installer for your operating system. Once downloaded, run the installer and follow the on-screen instructions to complete the installation process.
After installing Node.js, you will also have access to npm, which is the default package manager for Node.js. npm allows you to install and manage dependencies for your projects, making it easier to incorporate third-party libraries and tools into your SaaS dashboard.
To verify that Node.js and npm are installed correctly, you can open a terminal or command prompt and run the following commands:
node -v npm -v
These commands will display the version numbers of Node.js and npm, respectively. If you see the version numbers printed in the terminal, it means that Node.js and npm are installed correctly on your machine.
If you want our help in creating the app, you can always Hire React Native Developers here.
Creating Your React App
Now that you have Node.js and npm installed, we can create our React app using create-react-app. Create React App is a command-line tool that sets up a new React project with a basic file structure and build configuration. It also includes a development server that allows you to view your app in the browser during development. Additionally, we will need to import our logo into our project using regular javascript, which can be done by importing react from react and logo from our logo.
To create a new React app, open your terminal or command prompt and navigate to the directory where you want to create your project. Then, run the following command:
npx create-react-app my-saas-dashboard
This command will create a new directory called my-saas-dashboard
and set up a new React project inside it. It will also install all the necessary dependencies for the project, such as React, React DOM, and other packages required for React development.
Once the command completes, navigate to the my-saas-dashboard
directory:
cd my-saas-dashboard
Now you can open the project in your preferred code editor and start building your SaaS dashboard.
If you want our help in creating the app, you can always Hire React JS Developers here.
Integrating Firebase with Your React App
Next, we need to integrate Firebase with our React app. Firebase provides a JavaScript library that allows us to interact with Firebase services such as authentication real-time database, and storage.
To integrate Firebase, we will need to create a Firebase project and obtain the necessary configuration data. This data includes an API key, project ID, and other credentials that will allow our app to communicate with Firebase services.
Once we have the configuration data, we can initialize Firebase in our React app and start using Firebase services to build our SaaS dashboard.
Creating a Firebase Project
To create a Firebase project, you will need a Google account. If you don’t have one, you can create a new Google account for free.
Once you have a Google account, visit the Firebase website and sign in using your Google account credentials. After signing in, you will be taken to the Firebase console.
In the Firebase console, click on the “Add project” button to create a new project. Give your project a name, such as “My SaaS Dashboard”, and click on the “Continue” button.
On the next screen, you will have the option to enable Google Analytics for your project. You can choose to enable or disable Google Analytics based on your requirements.
After selecting your preference for Google Analytics, click on the “Create project” button to create your Firebase project. This may take a few seconds.
Once your project is created, you will be redirected to the project overview page. Here, you will find the configuration data for your Firebase project, including the API key, project ID, and other credentials.
Configuring Firebase Authentication
To configure Firebase Authentication, go to the Firebase console and select your project. In the side navigation, click on “Authentication” to access the authentication settings.
In the authentication settings, you can enable various sign-in methods for your SaaS dashboard. Firebase supports authentication methods such as email and password, Google sign-in, Facebook sign-in, and more.
Choose the sign-in methods that are appropriate for your SaaS dashboard and enable them in the Firebase console. This will allow your users to sign in to your dashboard using their preferred authentication method.
You can also configure additional security settings such as password complexity requirements, email verification, and account recovery options.
By configuring Firebase Authentication, you can add secure user authentication to your SaaS dashboard and ensure that only authorized users can access the dashboard’s features and functionality.
Setting Up Real-Time Database or Firestore in SaaS Dashboard
After configuring Firebase Authentication, you can set up the real-time database or Firestore for data storage in your SaaS dashboard.
Firebase offers two options for data storage: the real-time database and Firestore. The real-time database is a cloud-hosted NoSQL database that allows you to store and sync data in real time. Firestore, on the other hand, is a flexible and scalable NoSQL database that provides powerful querying capabilities and supports real-time updates.
To set up the real-time database or Firestore, go to the Firebase console and select your project. In the side navigation, click on “Database” to access the database settings.
Choose either the real-time database or Firestore, depending on your requirements. Follow the on-screen instructions to set up your database and configure the necessary security rules.
Once your database is set up, you can start using it to store and retrieve data for your SaaS dashboard. Firebase provides a JavaScript library that allows you to interact with the database and perform operations such as reading, writing, and updating data.
Designing the SaaS Dashboard Interface
The design of your SaaS dashboard interface plays a crucial role in providing a positive user experience. A well-designed dashboard interface should be intuitive, visually appealing, and easy to navigate.
To design your dashboard interface, you can use tools such as Figma, Sketch, or Adobe XD to create wireframes and prototypes. These tools allow you to visualize the layout, components, and overall design of your dashboard before implementing it in your React app.
Focus on creating a clean and organized design that makes it easy for users to find the information they need. Use a consistent color scheme, typography, and visual hierarchy to guide users through the dashboard and highlight important features.
Consider the user experience and ensure that the dashboard is responsive and accessible across different devices and screen sizes. Test your design with real users to gather feedback and make necessary improvements before implementing it in your React app.
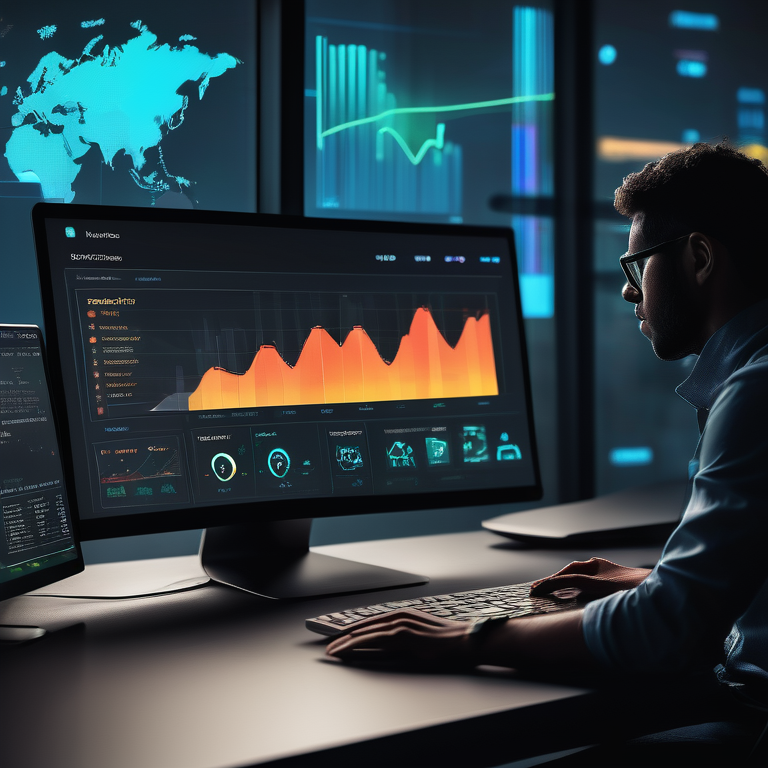
Sketching the Layout of SaaS Dashboard
Before diving into the implementation of your dashboard interface in React, it’s helpful to sketch out the layout and design of your dashboard. This can be done using tools like Figma, Sketch, or even pen and paper.
Start by creating a rough sketch of the overall layout of your dashboard. Consider the placement of different components, such as navigation menus, content areas, and widgets. Make sure to leave enough space for future expansion and additional features.
Once you have a rough layout, you can use design tools like Figma to create a more polished prototype of your dashboard. Figma allows you to create interactive prototypes that simulate the user experience of your dashboard. This can help you identify any usability issues or design flaws before implementing the interface in React. You can also check out the source code for this dashboard on my Github repository for reference.
Iterate on your design, gather feedback from users or stakeholders, and make any necessary adjustments Once you’re satisfied with the design, you can move on to implementing the dashboard interface in your React app.
Implementing Navigation Components
Navigation is a crucial component of any dashboard interface. It allows users to navigate between different sections and features of your SaaS dashboard.
In React, you can implement navigation using React components and routing libraries such as React Router. React Router provides a declarative way to define routes in your app and handle navigation between different components.
Start by designing your navigation menu or sidebar, considering the different sections and features of your dashboard. Create React components for each section and define the routes using React Router. Add appropriate links and navigation elements to your components to enable users to navigate between different sections.
Incorporate routing logic in your app’s main component or layout component to ensure that the correct components are rendered based on the current route. Use JSX syntax to define the routing and navigation elements in your React components.
By implementing navigation components in your React app, you can create a seamless and intuitive navigation experience for users of your SaaS dashboard.
Crafting the UI with React Components
To craft the UI with React components effectively, leverage the power of reusable components to streamline development. Utilize JSX to blend HTML structures with JavaScript seamlessly, enhancing component efficiency. Employ props to transfer data between components efficiently, ensuring a dynamic user interface. Implement CSS for styling to create visually appealing designs that resonate with users. Structure components logically within folders for organizational clarity, facilitating easy maintenance. By incorporating React functionalities like state and lifecycle methods, you can achieve interactive and responsive UI elements, improving user interaction and experience significantly.
Adding Authentication to Your SaaS Dashboard
Adding authentication to your SaaS dashboard is crucial for ensuring the security and privacy of user data. With Firebase Authentication, you can easily implement user authentication and management functionalities. Firebase Authentication supports various authentication methods, including email and password, Google, Facebook, and more. By integrating Firebase Authentication into your dashboard, you can provide secure access to authorized users and manage user sessions efficiently. This allows you to control the level of access and permissions for different users, ensuring a secure and personalized experience for each user of your SaaS dashboard.
An article on Fintech Security: AI Solutions for Safe Transactions might be of interest to you.
Setting Up User Authentication Flows
To set up user authentication flows in your SaaS dashboard, you need to define the authentication flow for different actions such as login, registration, and password management. With Firebase Authentication, you can easily handle these authentication flows, thanks to its user management features. For example, when a user wants to register, you can provide a registration form where they can enter their email and password. Firebase Authentication takes care of the validation, email verification, and storing the user credentials securely. Similarly, when a user wants to log in, you can provide a login form where they can enter their credentials, and Firebase Authentication handles the authentication process. Additionally, Firebase Authentication provides built-in functionality for password reset and recovery, making it easier for users to manage their passwords securely.
Managing User Sessions
Managing user sessions is essential for providing a seamless and secure experience in your SaaS dashboard. With Firebase Authentication, user sessions are automatically managed for you. When a user logs in, Firebase generates a unique authentication token that can be used to identify and authenticate the user in subsequent requests. This token is securely stored on the client side and sent with each request to the server to authenticate the user. Firebase handles the validation and expiration of the token, ensuring the security of the user’s session. Additionally, Firebase provides hooks and utilities to manage user sessions on the client side, allowing you to implement features such as automatic session persistence, session timeouts, and revoking user sessions if needed.
Connecting the SaaS Dashboard to Firebase Services
Firebase offers a wide range of services that can be seamlessly integrated into your SaaS dashboard. These services include Firebase Realtime Database, Firebase Firestore, Firebase Cloud Storage, Firebase Cloud Functions, and more. By connecting your dashboard to these Firebase services, you can leverage their powerful APIs to handle data storage, real-time data fetching, file uploads, serverless backend logic, and more. This allows you to build a scalable and robust SaaS dashboard without worrying about managing server infrastructure. Firebase’s real-time capabilities enable you to create dynamic and interactive dashboards that update in real-time as data changes, making it a valuable tool for businesses utilizing AI and machine learning platforms.
Fetching Data in Real-Time
Fetching data in real time is crucial for displaying up-to-date information in your SaaS dashboard. With Firebase Realtime Database or Firebase Firestore, you can easily fetch and sync data in real time between your dashboard and the database. Firebase provides powerful APIs that allow you to listen for changes in the data and automatically update the dashboard whenever there is a change. This enables you to create real-time updates, such as live chat, real-time analytics, real-time collaboration, and more. By leveraging Firebase’s real-time capabilities, you can create a highly interactive and responsive SaaS dashboard that provides a seamless user experience.
Handling Data Storage and Retrieval
Data storage and retrieval play a vital role in building a functional SaaS dashboard. With Firebase Realtime Database or Firebase Firestore, you can easily handle data storage and retrieval in your dashboard. Firebase provides a NoSQL database that allows you to store and query structured data in JSON format. You can create collections and documents to organize your data and perform CRUD operations using Firebase’s intuitive APIs. Whether you need to store user data, application settings, or any other type of data, Firebase offers a scalable and reliable solution. With Firebase’s offline capabilities, the data can be synced automatically when the user goes online, ensuring a seamless user experience even in challenging network conditions.
Deploying Your SaaS Dashboard
Deploying your SaaS dashboard is the final step in bringing your application to life. With Firebase Hosting, you can easily deploy your dashboard and make it live on the internet. Firebase Hosting provides a fast and secure hosting solution that automatically scales with your traffic. You can deploy your dashboard with a single command using the Firebase CLI, which takes care of building and deploying your application. Once deployed, your SaaS dashboard will be accessible to users worldwide, allowing them to interact with your application in real time.
Preparing for Deployment
Before deploying your SaaS dashboard, it’s essential to prepare your application for deployment. This involves optimizing the build process to ensure that your dashboard is efficient and performs well. You can use tools like Webpack or create-react-app to bundle and optimize your code for production. Minifying and compressing your assets can significantly reduce the file size and improve loading times. Additionally, you can leverage caching and content delivery networks (CDNs) to further optimize the performance of your dashboard. By following best practices for deployment and optimization, you can ensure that your SaaS dashboard is fast, reliable, and provides an excellent user experience.
Deploying to Firebase Hosting
Deploying your SaaS dashboard to Firebase Hosting is a straightforward process. After preparing your application for deployment, you can use the Firebase CLI to deploy your dashboard with a single command. The Firebase CLI automatically builds your application, optimizes the assets, and deploys it to Firebase Hosting. deployed, you can access your SaaS dashboard using the URL provided by Firebase Hosting. The Firebase console also provides a user-friendly interface to manage and monitor your deployed applications. You can easily update your dashboard, monitor usage, and control access to your application through the Firebase console.
Conclusion
In wrapping up the journey of building your SaaS Dashboard with React and Firebase, you have equipped yourself with valuable insights into the core objectives, tool selection, development environment setup, interface design, authentication implementation, data connectivity, and deployment strategies. By harnessing the power of React and Firebase, you are poised to create a dynamic, secure, and efficient dashboard that meets user expectations. Remember, continuous learning and adaptation are key to staying ahead in the tech landscape. If you’re ready to take your dashboard to the next level or have any questions, don’t hesitate to get in touch for further guidance and support.
Frequently Asked Questions
How to handle user permissions in the SaaS Dashboard?
To manage user permissions in your SaaS dashboard, utilize Firebase Authentication’s custom claims and roles. Assigning custom claims enables you to regulate user access. Firebase offers security rules for precise access control based on roles and data ownership. This enhances application security by restricting unauthorized actions and data access in the dashboard.
What are the best practices for SaaS Dashboard performance optimization?
Optimize your SaaS dashboard performance by following best practices like code splitting, lazy loading, and using memoization techniques in React. Utilize Firebase’s caching and CDN features to enhance loading times. Optimizing network requests, reducing render-blocking resources, and employing efficient CSS and JavaScript techniques will further boost your dashboard’s performance.
What are the benefits of using React for building a SaaS Dashboard?
React offers benefits for SaaS dashboard development like component-based architecture, virtual DOM, and efficient rendering. Its declarative syntax allows for easier code maintenance and reuse. Performance optimizations like the virtual DOM lead to faster updates and a more responsive user interface.
How does Firebase contribute to the development of a SaaS Dashboard?
Firebase offers tools for developing SaaS dashboards, including real-time data sync, authentication, storage, and serverless functions. These features help build scalable dashboards without infrastructure concerns, ensuring your dashboard can grow with your business.