Refactoring is super important for any developer who wants to keep their code clean, easy to manage, and ready to grow. It’s all about making little tweaks here and there in the codebase. These changes help make everything inside work better, look nicer, and run smoother without messing with how things work on the outside. For folks working with PHP and other programming languages like JavaScript – a place where codes can get really tangled up over time – getting good at refactoring is key to saving a lot of time and effort. In fact, functions like map and reduce, commonly used in JavaScript, can dramatically improve the readability of your code.
In this blog post, we’re going through 10 awesome tips that’ll seriously level up your PHP coding game. We’ve got a bunch of strategies lined up that will not only boost the quality but also make sure your php code stays easy to handle.
We’ll talk about sticking to one job per class (that’s what they call Single Responsibility Principle), making boolean expressions easier on the brain, choosing composition instead of inheritance when you can, and even trying out something called the Strangler Fig Pattern among other cool tricks. All these are aimed at fixing common headaches in coding projects and pushing up the standard of your PHP work.
No matter if you’re pretty experienced or just beginning with PHP development , these pointers are bound to give you some fresh ideas on how to refine those refactoring skills further . Let’s jump right into it!
10 PHP Refactoring Tips and Tricks for Meticulous Developers
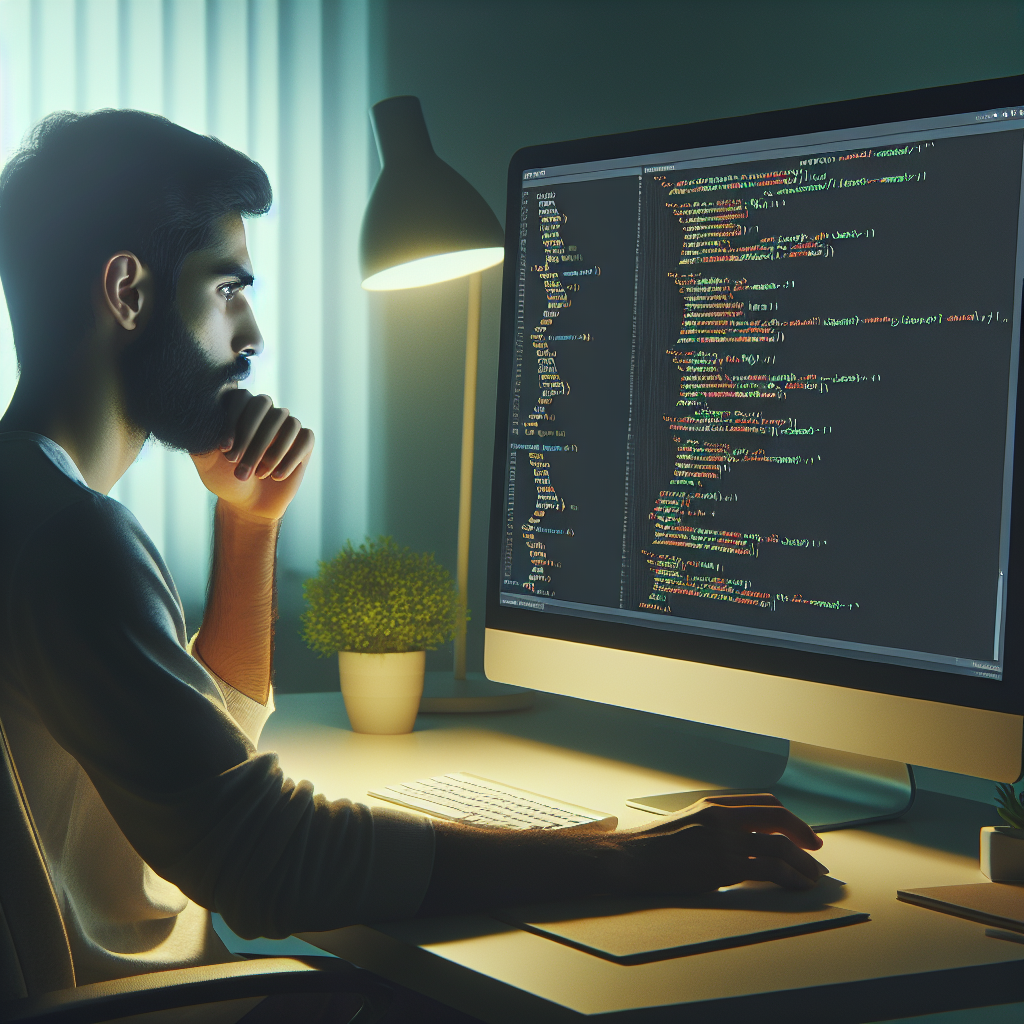
Refactoring plays a crucial role in creating software, and this holds true for PHP developers as well. Below are ten transformative tips and tricks aimed at helping detail-oriented developers enhance their PHP refactoring abilities, including the use of tools like Rector. These suggestions touch on everything from the principles of clean code to how your code is organized, including patterns you can use when refactoring in the PHP programming language. By adopting these strategies, developers will find they’re able to greatly boost the quality, readability, and maintainability of their php code.
1. Embrace the Single Responsibility Principle for Cleaner Code
The Single Responsibility Principle (SRP) is a fundamental principle of clean code that states that a class or module should have only one reason to change. By adhering to this principle, you can ensure that each class or module is responsible for a single, well-defined task, making your codebase more maintainable and easier to understand.
When refactoring your PHP code, analyze each class or module and determine if it has multiple responsibilities. If so, consider breaking it down into smaller, more focused components. This allows you to isolate specific functionality and make your code more modular. By embracing SRP, you can create cleaner, more maintainable code that is easier to test and debug.
SRP Before Refactoring | SRP After Refactoring |
A class responsible for user authentication and data validation. | A separate class for user authentication and another for data validation. |
2. Simplify Your Boolean Expressions for Better Readability
Boolean expressions can quickly become complex and difficult to understand, especially when they involve multiple conditions and logical operators. To improve the readability of your code, consider simplifying your boolean expressions.
One way to do this is by extracting complex conditions into separate variables with meaningful names. This allows you to break down the logic and make it easier to understand at a glance. Additionally, consider using helper functions or methods to encapsulate complex boolean logic.
Complex Boolean Expression Before Refactoring | Simplified Boolean Expression After Refactoring |
if (a && (b | a && b && c |
Check out an article on Optimizing JavaScript Performance: Tips and Tricks.
3. Utilize Composition Over Inheritance to Enhance Flexibility
Inheritance can be a powerful tool in object-oriented programming, but it can also lead to tight coupling and inflexible code. Instead, consider utilizing composition over inheritance to enhance flexibility and modularity in your codebase.
Composition involves creating classes that are composed of other classes or modules, rather than relying on inheritance. This allows you to easily change and modify behavior by swapping out components at runtime.
By favoring composition over inheritance, you can achieve a more flexible and maintainable codebase. Each component can be developed independently and easily tested in isolation. This approach also promotes code reusability and reduces the risk of introducing unwanted dependencies.
Inheritance Before Refactoring | Composition After Refactoring |
class Car extends Vehicle { … } | class Car { private $vehicle; … } |
4. Implement Naming Conventions for Clarity and Consistency
Consistent and meaningful names are crucial for writing clean and readable code. By implementing naming conventions, you can improve code clarity and maintainability, making it easier for other developers to understand and work with your code.
Consider using descriptive and self-explanatory names for variables, functions, classes, and other elements in your code. This ensures that the purpose and functionality of each element are clear at a glance. Additionally follow a consistent naming convention throughout your codebase to maintain uniformity and reduce confusion.
Inconsistent Naming Before Refactoring | Consistent Naming After Refactoring |
$x = 10; $y = calculateSum($x); | $number = 10; $sum = calculateSum($number); |
5. Break Down Large Classes with the Extract Class Method
Large classes can become difficult to understand, maintain, and test. To improve the modularity and readability of your code, consider breaking down large classes into smaller, more focused components using the Extract Class method.
Identify distinct responsibilities within a large class and extract them into separate classes. This allows you to encapsulate related behavior and create more cohesive and modular code. By doing so, you can also reduce code duplication and improve code reusability.
Large Class Before Refactoring | Extracted Classes After Refactoring |
class User { … } | class User { … } class Address { … } |
Here’s an article on Cypress vs Playwright: Which Automated Testing Tool is Right for You?
6. Refactor by Extracting Methods for Improved Modularity
Extracting methods is a powerful PHP refactoring technique that allows you to improve the modularity and readability of your code. By identifying repetitive or complex logic, you can extract it into separate methods, making your code more modular and easier to understand.
When extracting methods, choose meaningful names that accurately describe the functionality of the extracted code. This makes your code more self-explanatory and reduces the need for comments. Additionally, consider passing parameters to the extracted methods to make them more generic and reusable.
Repetitive Code Before Refactoring | Extracted Methods After Refactoring |
if (a > b) { … } else { … } | if (isGreater(a, b)) { … } else { … } |
7. Replace Nested Conditionals with Guard Clauses
Nested conditionals can make code difficult to follow and understand. To simplify your code and improve its readability, consider replacing nested conditionals with guard clauses.
Guard clauses allow you to handle exceptional cases or preconditions at the beginning of a method or function. By checking for invalid or unexpected conditions early on, you can exit the method or function early and avoid unnecessary nesting.
This technique improves code clarity and reduces cognitive load by removing unnecessary indentation and minimizing the number of code paths to follow.
Nested Conditionals Before Refactoring | Guard Clauses After Refactoring |
function calculatePrice($quantity, $price) { if ($quantity > 0) { if ($price > 0) { … } } } | function calculatePrice($quantity, $price) { if ($quantity <= 0) { return 0; } if ($price <= 0) { return 0; } … } |
8. Use Polymorphism to Avoid Switch Statements
Switch statements can become cumbersome and difficult to maintain, especially when dealing with multiple cases. To improve the flexibility and maintainability of your code, consider using polymorphism instead.
Polymorphism allows you to define common behaviors and interfaces for different classes, enabling you to handle different cases without resorting to switch statements. By leveraging inheritance and interfaces, you can write cleaner and more extensible code that is easier to test and maintain.
This approach also reduces the risk of introducing bugs or forgetting to handle specific cases when modifying or extending your codebase.
Switch Statement Before Refactoring | Polymorphism After Refactoring |
switch ($type) { case ‘circle’: … break; case ‘rectangle’: … break; } | interface Shape { public function calculateArea(); } class Circle implements Shape { public function calculateArea() { … } } class Rectangle implements Shape { public function calculateArea() { … } } |
9. Leverage Dependency Injection for Easier Maintenance
Dependency injection is a powerful technique that promotes loose coupling and improves the maintainability of your code. By injecting dependencies rather than directly instantiating them, you can easily replace or modify components without impacting the rest of your codebase.
When applying dependency injection, identify the dependencies of a class or module and pass them as parameters or inject them through a container or framework. This allows you to decouple your code and make it more modular, reusable, and testable.
By leveraging dependency injection, you can easily swap out components, mock dependencies for testing purposes, and improve the overall flexibility and maintainability of your code.
Direct Instantiation Before Refactoring | Dependency Injection After Refactoring |
class UserService { private $userRepository; public function __construct() { $this->userRepository = new UserRepository(); } } | class UserService { private $userRepository; public function __construct(UserRepository $userRepository) { $this->userRepository = $userRepository; } } |
An article on JavaScript: Is It Losing Its Charm? might be of interest to you.
10. Apply the Strangler Fig Pattern for Incremental Refactoring
The Strangler Fig Pattern is a powerful technique for refactoring large and complex codebases incrementally. This pattern involves gradually replacing or “strangling” the old code with new and improved components until the entire codebase has been refactored.
To apply the Strangler Fig Pattern, identify specific modules or functionalities within your codebase that require PHP refactoring. Create new components that provide the same functionality as the old code and gradually replace the old code with the new components.
This approach allows you to refactor your codebase incrementally without disrupting the overall functionality of your application. It reduces the risks associated with large-scale refactoring and enables you to tackle refactoring in manageable chunks.
Old Code Before Refactoring | Strangler Fig Pattern After Refactoring |
Old monolithic codebase | New components gradually replace the old codebase over time |
Deep Dive into PHP Refactoring Techniques
After going through the 10 transformative tips and tricks for PHP refactoring, it’s time to delve deeper into the essential techniques and ideas that make up PHP refactoring. With a good grasp of these methods, you’ll be in a great position to use them well in your projects, making you a more careful developer.
Understanding the Strangler Fig Pattern in PHP Refactoring
The Strangler Fig Pattern is a cool way developers use in PHP to update old code bit by bit with new, better parts. It’s named after the strangler fig tree because it wraps around and slowly takes over another tree.
With this pattern, developers pick out certain parts or functions in the old code that need updating and make new components for them. These new bits start doing what the old ones did but in a newer, better way while making sure everything else still works just fine.
By using the Stranger Fig Pattern, folks can tidy up messy codes step by step without causing big problems. This method comes in really handy when dealing with outdated codes that need some freshening up.
The Power of Static Analysis Tools in PHP Code Maintenance
Static analysis tools are super important for keeping PHP code in good shape. They look at the code without actually running it, pointing out possible bugs, performance problems, and other issues that don’t smell right. With these tools, developers can spot mistakes early on, which helps make sure the quality of the PHP code is top-notch and cuts down on technical debt.
For instance, there’s this tool called PHPStan that goes through your code to find things like type errors or places where you’ve used a variable that doesn’t exist yet. It also looks for parts of your code that aren’t being used anywhere (dead code). Then we have Psalm; it’s another tool but focuses more on finding type errors and guessing types in your PHP codes. These tools are great because they show developers where their codes might be going wrong and offer tips to fix them.
But static analysis isn’t everything when it comes to taking care of our php codesbase . Code reviews play a big part too since they let devs share what they know with each other while spotting potential problems together so everyone can learn how to do better next time around improving overall code quality . And then there’s automated testing – think unit tests or integration tests – which makes sure everything works as expected by catching any slip-ups before they become bigger headaches later.
So yeah combining static analysis with stuff like reviewing each others’ work plus doing lots of automatic checks means we get really solid php PHP coding practices helping keep up high standards across our projects.
Advanced PHP Refactoring: The Role of Automated Tests
Automated tests are super important when it comes to tweaking and improving PHP code. They make sure everything still works right after you’ve made changes, catching any slip-ups or unexpected results from those tweaks.
With these tests ready to go, developers can dive into fixing up the code without worrying about messing things up. This freedom means they can do big stuff like rearranging how the code is put together, making it run faster, or even just making it easier to read—all without risking breaking what was already working fine.
When we talk about automated testing for this kind of work, there are a few different kinds you might use: unit tests that look at each piece of your code on its own; integration teststhat see how those pieces work together; and functional tests that check if the whole thing does what users expect.
Mixing automated testing with other smart ways to tweak your coding not only makes your PHP projects better but also cuts down on technical debt—that’s basically the extra work that piles up when quick fixes get used instead of proper solutions—keeping everything easy to handle and build on in the future.
Overcoming Common PHP Refactoring Challenges
When you’re working on refactoring code, it’s not always smooth sailing. In this part, we’ll dive into a few usual hurdles that pop up while going through the refactoring process and share some advice on how to get past them. With these tips, tackling the challenges of PHP refactoring, including identifying and fixing flaws in its internal structure, should become a bit easier for you.
Handling Legacy Code: Strategies and Best Practices
Legacy code is basically the old stuff in programming that’s tough to deal with, change, or even understand. When you try to tidy up or refactor this legacy code, it can get pretty tricky because it’s complex and often doesn’t come with instructions on how it works. But there are ways to tackle these issues.
For starters, instead of trying to overhaul all the existing code at once, making small changes bit by bit can be a smarter move. This method lets programmers make improvements slowly but surely without messing things up too much. Before diving into any changes, it’s crucial to really get what the current code does and its purpose.
On top of that, there are some cool tools and frameworks out there like Laravel which help a lot when dealing with legacy code during the refactoring process. These tools have special features designed for working on old codes such as creating new parts automatically, updating databases smoothly and checking your work through automated tests which makes cleaning up outdated codes not only easier but also more effective.
Refactoring in a Team: Coordinating Efforts and Best Tools
When a team works on tidying up code, it’s really important for everyone to talk and work together well. This makes sure we’re all heading in the same direction. It’s key to have clear rules about how to clean up the code and make sure everyone sticks to them.
By using tools like Git, which lets us keep track of different versions of our work, we can all work on our own parts without stepping on each other’s toes. It also keeps a record of what changes were made, so if something goes wrong, it’s easy to fix.
For cleaning up code, there are special programs called IDEs (like PhpStorm) that come with handy features. They check your code automatically, help you move around in it easily and even give suggestions on making it better. These programs make the whole PHP refactoring process smoother and help keep the quality of our code top-notch.
Balancing PHP Refactoring with Feature Development
When building software, it’s crucial to balance improving existing code (refactoring) with adding new features. High-quality code is essential, but meeting deadlines by introducing new features is also important.
One way to handle this is by setting aside specific times just for cleaning up and improving the code. This means developers get a chance to focus on tidying up without worrying about getting new features out. By planning these cleanup sessions carefully, it’s possible to keep both the quality of the codebase high and keep adding cool new things.
Another smart move is to clean up a little bit at a time while you’re already working on adding those features. Instead of seeing cleaning up as something extra or separate, it becomes part of everyday work. This keeps everything running smoothly and makes sure that updating doesn’t slow down bringing in exciting updates.
Case Studies: Successful PHP Refactoring Projects
In this part, we’re diving into stories about PHP refactoring projects that turned out really well. We’ll talk about how the refactoring process made things better and what good stuff came from it.
From Monolithic to Microservices: A PHP Refactoring Journey
The transition from a monolithic architecture to a microservices architecture is a common refactoring journey. This involves breaking down a monolithic application into smaller, decoupled services that can be developed and deployed independently.
Before Refactoring | After Refactoring |
Monolithic application with tightly coupled components | Decoupled microservices with independent development and deployment |
Single codebase and database | Separate codebases and databases for each microservice |
Scaling the entire application as a whole | Scaling individual microservices as needed |
Difficult to add new features without affecting the entire application | Flexibility to add new features to specific microservices |
Complex deployment process | Simplified deployment with containerization and orchestration |
By refactoring a monolithic PHP application into microservices, developers can achieve greater scalability, flexibility, and maintainability. Each microservice can be developed, tested, and deployed independently, allowing for faster development cycles and easier maintenance.
Check out an article on Monolithic vs Microservices: Which is Better for You?
Enhancing Performance and Scalability Through Strategic Refactoring
By smartly tweaking the codebase, we can make PHP applications run faster and handle more users. This means finding where the app slows down and fixing those spots to speed things up.
Improving database performance involves optimizing data retrieval by organizing it better (indexing), using smarter queries, and implementing caching for faster results. Streamlining code by eliminating unnecessary steps and enhancing calculations further boosts performance.
For an application to grow smoothly without hiccups when more people start using it involves changing its structure a bit. Think about dividing it into smaller parts (microservices). Spread requests evenly (load balancing). Store data temporarily for faster access next time (use caching). By focusing on these aspects during changes, developers guarantee that when demand increases, the application remains responsive.
Conclusion
In PHP refactoring, follow 10 helpful tips to improve code readability and maintenance. Ensure each part serves one purpose and use techniques like Dependency Injection for simplicity. These methods streamline the codebase, leading to smoother operations. Mastering these strategies enhances code quality and prepares for future enhancements. Learning PHP refactoring techniques brings significant improvements to your development workflow. And if you’re looking for some advice or want to dig deeper, don’t hesitate to reach out for professional help in getting good at PHP refactoring.
Frequently Asked Questions
What are the first steps in PHP refactoring?
When you start to tidy up PHP code, the first thing to do is get a good grasp of what’s already there. Look for parts that aren’t up to scratch and decide what you want to achieve by making changes. It’s crucial to figure out which bits need fixing first based on how much they’ll improve the overall quality of the code. Dealing with technical debt is also key so that your code stays easy to work with down the line.
How do you prioritize refactoring tasks?
When it comes to deciding which refactoring tasks to tackle first, you’ve got to think about how much they’ll help improve the code quality, how serious the technical debt is, and what good could come out of each task. It’s smart to begin with small changes that are easy to handle and then slowly move on to bigger challenges in refactoring. This way, you can keep adding new features while also making your code better.
Can PHP refactoring impact the user experience?
Refactoring is all about making the inside of an app better and improving how well it’s put together. But, if we’re not careful or skip testing, refactoring might mess up how the app works for users by bringing in bugs or messing with its external behavior. With this in mind, it’s crucial to really check over any changes made during PHP refactoring to keep everything running smoothly for those using the application.